kcl-samples → flange-with-patterns →
flange-with-patterns
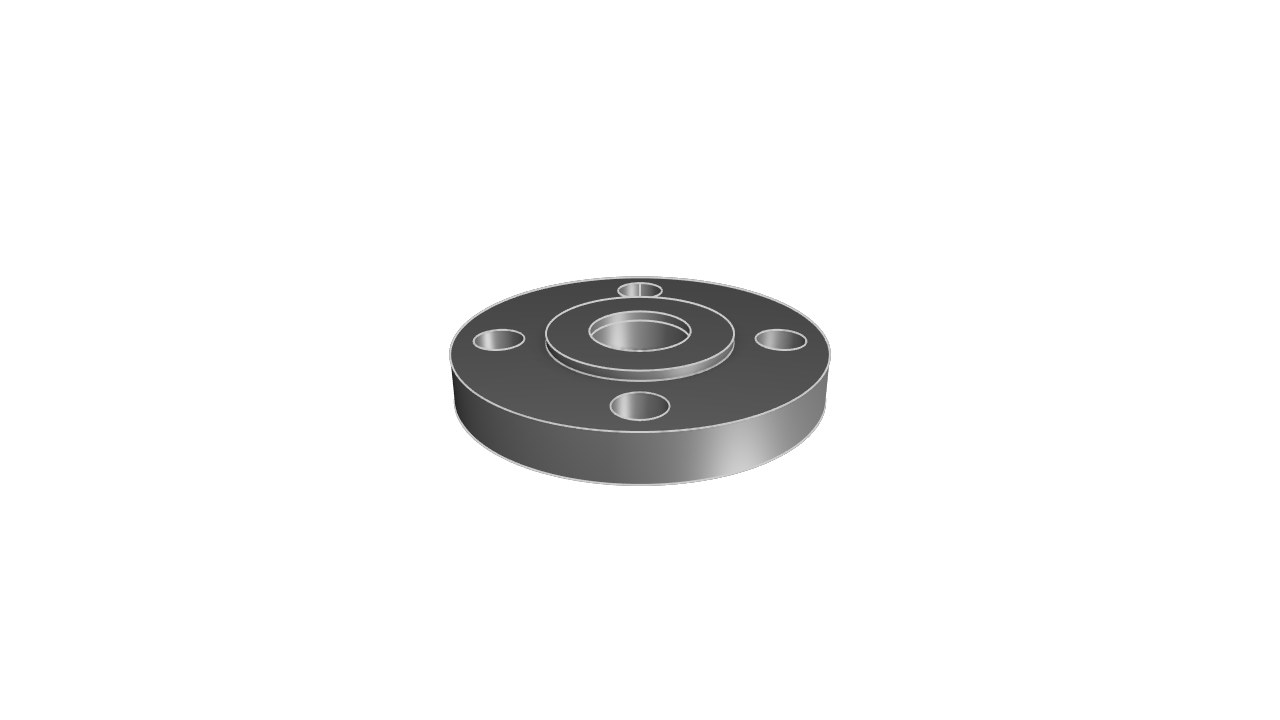
KCL
// Flange
// A flange is a flat rim, collar, or rib, typically forged or cast, that is used to strengthen an object, guide it, or attach it to another object. Flanges are known for their use in various applications, including piping, plumbing, and mechanical engineering, among others.
// Define constants
mountingHoleDia = .625
baseDia = 4.625
pipeDia = 1.25
thickness = .625
totalThickness = 0.813
topTotalDiameter = 2.313
bottomThickness = 0.06
bottomTotalDiameter = 2.5
mountingHolePlacementDiameter = 3.5
baseThickness = .625
topTotalThickness = totalThickness - (bottomThickness + baseThickness)
holeLocator = baseDia - 8
nHoles = 4
// Add assertion so nHoles are always greater than 1
assertGreaterThan(nHoles, 1, "nHoles must be greater than 1")
// Create the circular pattern for the mounting holes
circles = startSketchOn('XY')
|> circle({
center = [mountingHolePlacementDiameter / 2, 0],
radius = mountingHoleDia / 2
}, %)
|> patternCircular2d({
arcDegrees = 360,
center = [0, 0],
instances = nHoles,
rotateDuplicates = true
}, %)
// Create the base of the flange and add the mounting holes
flangeBase = startSketchOn('XY')
|> circle({
center = [0, 0],
radius = baseDia / 2
}, %)
|> hole(circles, %)
|> hole(circle({
center = [0, 0],
radius = pipeDia / 2
}, %), %)
|> extrude(baseThickness, %)
// Plane for top face
topFacePlane = {
plane = {
origin = { x = 0, y = 0, z = baseThickness },
xAxis = { x = 1, y = 0, z = 0 },
yAxis = { x = 0, y = 1, z = 0 },
zAxis = { x = 0, y = 0, z = 1 }
}
}
// Create the extrusion on the top of the flange base
topExtrusion = startSketchOn(topFacePlane)
|> circle({
center = [0, 0],
radius = topTotalDiameter / 2
}, %)
|> hole(circle({
center = [0, 0],
radius = pipeDia / 2
}, %), %)
|> extrude(topTotalThickness, %)
// Create the extrusion on the bottom of the flange base
bottomExtrusion = startSketchOn("XY")
|> circle({
center = [0, 0],
radius = bottomTotalDiameter / 2
}, %)
|> hole(circle({
center = [0, 0],
radius = pipeDia / 2
}, %), %)
|> extrude(-bottomThickness, %)